If you're preparing for a Java interview, there’s one area you absolutely can’t ignore—Java operators. These little symbols might seem basic at first, but they play a significant role in how Java works under the hood. Whether you're a beginner or an experienced developer brushing up for an interview, mastering these operators is crucial.
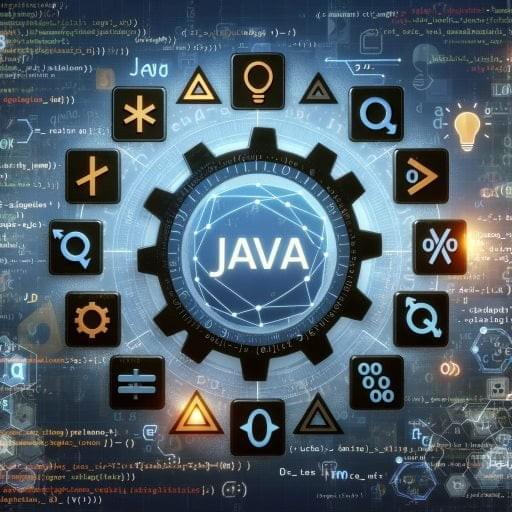
In simple terms, Java operators are special symbols used to perform operations on variables and values. They’re the backbone of any logical or mathematical operation you'll perform in Java. From basic arithmetic to complex logical comparisons, operators are everywhere in your code. Let's walk through the essential ones every Java developer should know.
1.
Arithmetic Operators
These are the basic operators used to perform mathematical calculations like addition, subtraction, multiplication, division, and modulus.
- Addition (+): Adds two values.
- Subtraction (-): Subtracts one value from another.
- Multiplication (*): Multiplies two values.
- Division (/): Divides one value by another.
- Modulus (%): Finds the remainder when one value is divided by another.
You’ll often encounter Java interview questions asking you to manipulate numbers, so having a firm understanding of arithmetic operators is vital.
2.
Relational Operators
Relational operators are used to compare two values. The result of a comparison using these operators is always a boolean (true or false).
- Equal to (==): Checks if two values are equal.
- Not equal to (!=): Checks if two values are not equal.
- Greater than (>): Checks if the first value is greater than the second.
- Less than (<): Checks if the first value is less than the second.
- Greater than or equal to (>=): Checks if the first value is greater than or equal to the second.
- Less than or equal to (<=): Checks if the first value is less than or equal to the second.
In an interview, you might be asked to compare variables or implement logical conditions. Understanding how these operators work is key to answering those Java interview questions correctly.
3.
Logical Operators
Logical operators are used to combine multiple conditions. They’re particularly important when you're writing if-else conditions or loops.
- AND (&&): Returns true if both conditions are true.
- OR (||): Returns true if at least one condition is true.
- NOT (!): Inverts the result of a condition.
Expect to see these operators in Java interview questions that deal with decision-making or multiple conditions. You might have to check if a number is both positive and even, for example, and this is where logical operators will help.
4.
Assignment Operators
These operators are used to assign values to variables. The most common one is the equals sign (=), but there are compound assignment operators that combine arithmetic with assignment.
- Simple assignment (=): Assigns the right-hand value to the left-hand variable.
- Addition assignment (+=): Adds the right-hand value to the left-hand variable and assigns the result.
- Subtraction assignment (-=): Subtracts the right-hand value from the left-hand variable and assigns the result.
Understanding how assignment works, especially in conjunction with arithmetic operations, can save you in tricky interview scenarios.
5.
Bitwise Operators
These are more advanced operators that work at the bit level. They’re often used in scenarios requiring low-level data manipulation, and while they’re not as common as arithmetic or logical operators, they can show up in Java interview questions for more experienced roles.
- Bitwise AND (&): Compares each bit of two values and returns 1 if both bits are 1.
- Bitwise OR (|): Compares each bit of two values and returns 1 if at least one of the bits is 1.
- Bitwise XOR (^): Compares each bit of two values and returns 1 if one of the bits is 1 and the other is 0.
While these operators may seem niche, understanding them can impress interviewers, especially when tackling technical challenges or optimizations.
Why Mastering Java Operators is Essential for Interview Success
Java operators are not just foundational—they’re frequently featured in coding challenges, algorithms, and problem-solving scenarios in interviews. Interviewers often ask candidates to solve problems involving variables, data manipulation, and control flow, all of which depend heavily on a solid understanding of operators.
Whether you're asked to implement logic for sorting numbers, compare datasets, or write complex conditions, operators will be at the heart of your solution. Brushing up on these concepts will not only help you tackle basic Java interview questions, but it will also give you confidence to take on more advanced challenges.
Practice Makes Perfect
The best way to get comfortable with Java operators is through practice. Write small programs that use each operator, solve coding challenges, and go through previous Java interview questions related to operators. The more hands-on experience you get, the more likely you are to ace your next interview.
Final Thoughts
Mastering Java operators is a must for anyone preparing for a technical interview. From basic arithmetic to logical decision-making, operators are crucial tools in any Java developer's toolkit. Make sure to spend time getting familiar with how they work, and you'll be better prepared to handle whatever interview questions come your way!