Today, we're going to dive deep into two important concepts: Armstrong numbers and prime numbers. Whether you're new to programming or looking to expand your skills, understanding these fundamental concepts will greatly enhance your Python knowledge. By the end of this blog, you'll be able to write Python programs to identify Armstrong and prime numbers with confidence.
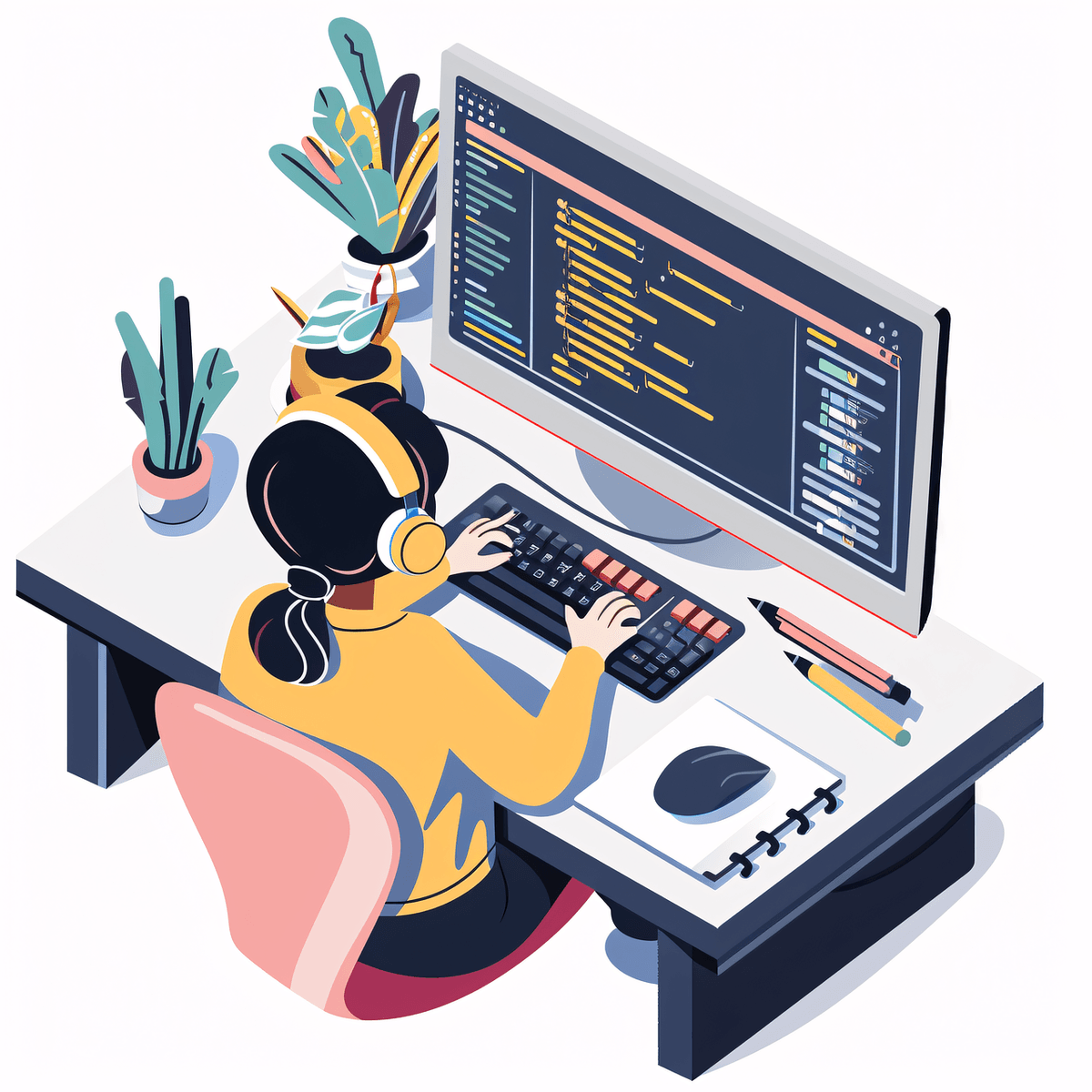
Before we dive into the code, let's clarify what Armstrong and prime numbers are and why they matter in programming.
An Armstrong number (also known as a narcissistic number) is a number that is equal to the sum of its own digits each raised to the power of the number of digits. For example, 153 is an Armstrong number because
13+53+33=153
On the other hand, a prime number is a number greater than 1 that has no positive divisors other than 1 and itself. For instance, 2, 3, 5, and 7 are prime numbers.
Understanding these concepts not only sharpens your programming skills but also lays a solid foundation for tackling more complex algorithms in Python.
Introduction to Armstrong Numbers
Definition of Armstrong Numbers
Let's break down Armstrong numbers in Python further. Imagine a number that seems to be in love with itself—it’s a number that equals the sum of its digits each raised to the power of the number of digits it has. For example, 153 is an Armstrong number because 13+53+33=153
Mathematical Explanation
To determine if a number is an Armstrong number, you can follow a straightforward formula. It involves converting the number to a string to easily iterate over its digits, calculating the sum of these digits raised to the power of the number of digits, and comparing this sum to the original number. This approach not only enhances our coding skills but also deepens our understanding of the underlying logic.
Writing a Python Program to Check Armstrong Numbers
Now, let's write some Python code to check if a number is an Armstrong number. Don't worry if you're not familiar with Python syntax—we'll explain everything step by step.
def is_armstrong_number(num):
num_str = str(num)
num_len = len(num_str)
sum_of_powers = sum(int(digit) ** num_len for digit in num_str)
return num == sum_of_powers
# Let's test our function!
number = int(input("Enter a number: "))
if is_armstrong_number(number):
print(f"{number} is an Armstrong number.")
else:
print(f"{number} is not an Armstrong number.")
In this code:
- We define a function is_armstrong_number that takes a number num as input.
- Convert the number to a string to easily iterate over its digits.
- Calculate the sum of each digit raised to the power of the number of digits.
- Compare the calculated sum with the original number to determine if it’s an Armstrong number.
Feel free to try different numbers and see the results for yourself!
Introduction to Prime Numbers
Definition of Prime Numbers
Now, let's shift our focus to prime numbers—a concept that's both simple and profound. A prime number in Python is a number greater than 1 that has no positive divisors other than 1 and itself. For example, 2, 3, 5, and 7 are prime numbers.
Mathematical Explanation
To determine if a number is prime, we need to check if it has divisors other than 1 and itself. This involves testing divisibility from 2 up to the square root of the number. This approach efficiently determines whether a number is prime and demonstrates how mathematical concepts can be applied in programming.
Writing a Python Program to Check Prime Numbers
Let's now write Python code to check if a number is prime. Python makes it straightforward to implement this logic.
def is_prime_number(num):
if num <= 1:
return False
for i in range(2, int(num ** 0.5) + 1):
if num % i == 0:
return False
return True
# Let's test our function!
number = int(input("Enter a number: "))
if is_prime_number(number):
print(f"{number} is a prime number.")
else:
print(f"{number} is not a prime number.")
In this code:
- We define a function is_prime_number that takes a number num as input.
- Check if the number is less than or equal to 1 (not prime).
- Iterate through numbers from 2 to the square root of num to check for divisibility.
- Return True if no divisors other than 1 and num are found; otherwise, return False.
Combining Armstrong and Prime Number Checks
Creating a Unified Program
Now, let’s take our learning a step further by creating a single program that can check both Armstrong and prime numbers. This demonstrates how versatile Python can be in handling different algorithms within the same script.
Code Example for Combined Check
def is_armstrong_number(num):
num_str = str(num)
num_len = len(num_str)
sum_of_powers = sum(int(digit) ** num_len for digit in num_str)
return num == sum_of_powers
def is_prime_number(num):
if num <= 1:
return False
for i in range(2, int(num ** 0.5) + 1):
if num % i == 0:
return False
return True
# Let's test our unified program!
number = int(input("Enter a number: "))
if is_armstrong_number(number):
print(f"{number} is an Armstrong number.")
else:
print(f"{number} is not an Armstrong number.")
if is_prime_number(number):
print(f"{number} is a prime number.")
else:
print(f"{number} is not a prime number.")
In this combined code:
- We have both is_armstrong_number and is_prime_number functions defined.
- We input a number and check if it is both an Armstrong and a prime number.
This unified program showcases the flexibility of Python in handling different algorithms efficiently.